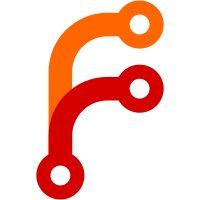
git-svn-id: svn+ssh://atelier.inf.usi.ch/home/bevilj/group-1@155 a672b425-5310-4d7a-af5c-997e18724b81
57 lines
No EOL
1.9 KiB
HTML
57 lines
No EOL
1.9 KiB
HTML
---
|
|
layout: page
|
|
category-page: scripts
|
|
category-title: Scripting
|
|
tags: loop for while range do
|
|
author: Marco Tereh
|
|
title: Loops
|
|
---
|
|
|
|
A very useful feature for scripting is loops; that is, the ability to write a repeating piece of code.<br>
|
|
Loops take this form:
|
|
<pre>
|
|
[condition] do
|
|
[repeated code]
|
|
done
|
|
</pre>
|
|
|
|
|
|
In particular, there are two types of loops, for loops and while loops.<br>
|
|
For loops are written like this:
|
|
<pre>
|
|
for [variable] in [list] do
|
|
[code]
|
|
done
|
|
</pre>
|
|
Their function is to <i>iterate</i> over a list. The list can take various forms, but the variable will always be
|
|
one of its elements. In particular, it will be the first element of the list on the first iteration and advance by
|
|
one element in every iteration after that. Most importantly, it can be accessed from inside the repeating code.<br>
|
|
<br>
|
|
The most basic kind of list is a numerical range, written like this:
|
|
<pre>
|
|
for i in {1..10}; do
|
|
[code]
|
|
done
|
|
</pre>
|
|
<code>{1..10}</code> means "the list of every number between 1 and 10 (inclusive)".
|
|
<code>i</code> is the variable that will hold one of these numbers in every iteration.
|
|
Here's a simple example of how to use it:
|
|
<pre>
|
|
for i in {1..10}; do
|
|
echo $i;
|
|
done
|
|
</pre>
|
|
<!-- TODO: insert the correct link here once the variables page is up -->
|
|
<a href="#"><code>$i</code> is how you access the variable i</a>.
|
|
Ranges can also count backward like this: <code>{10..1}</code>.
|
|
Another way to say what <code>{1..10}</code> means is "The numbers between 10 and 1, <i>in increments of 1</i>".
|
|
What if you want increments of two, though? What if you want every <i>even</i> number in a range?<br>
|
|
Numerical ranges also allow you to specify the size of the increments or decrements between each element in the list,
|
|
more generally called the <i>step size</i>.
|
|
Here's an example:
|
|
<pre>
|
|
for i in {0..10..2}; do
|
|
echo $i;
|
|
done
|
|
</pre>
|
|
<!-- UNFINISHED --> |